Chapter 2 Python Revision Tour II
Class 12 Computer Science Sumita Arora Book Solutions
Type A: Short Answer Questions / Conceptual Questions – Python Revision Tour II
Question – 1:
What is the internal structure of Python strings ?
Answer: In Python, Strings are stored by storing each character separately in contiguous locations.
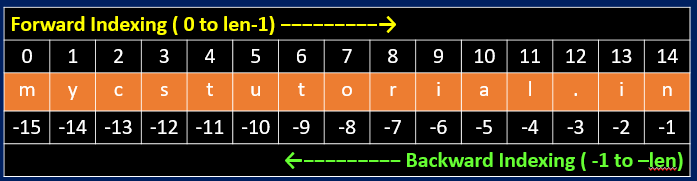
The characters of strings are given two-way indices – forward direction 0, 1, 2, 3 .. size-1, and backward direction -1, -2, …, -size.
Question – 2:
Write a python script that traverses through an input string and prints its characters in different lines – two characters per line.
Answer: Python Code-
string = input("Enter a String : ")
for j in range(0 , len(string), 2):
print(string[j : j+2])
Output:
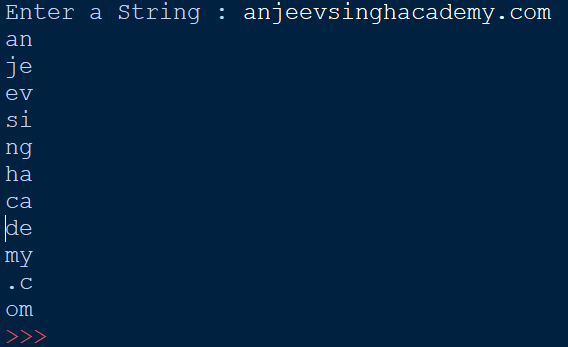
Question – 3:
Discuss the utility and significance of Lists, briefly.
Answer: List is a standard data type of Python that can store a sequence of values belonging to any type. It is depicted through square brackets.
Lists are mutable, which means we can change the element of a list in place.
Lists play a vital role in implementing stacks and queues in Python.
Due to its mutability feature, you can use it at several places where you need to make changes at run time.
Question-4:
What do you understand by mutability ? What does “in place” task mean ?
Answer: Mutability means you can change the values of elements in place. In-place means at the same memory location.
Generally in Python, when you change the value of an immutable variable, it starts pointing to another memory location, which means changes are not done in the same memory.
While in the case of mutable variables, changes take place at the same memory, it does not start pointing to another memory location.
Question – 5:
Start with the List = [8, 9, 10]. Do the following operation
- (a) Set the second entry (index 1) to 17.
- (b) Add 4, 5, and 6 to the end of the list.
- (c) Remove the first entry from the list.
- (d) Sort the list.
- (e) Double the list.
- (f) Insert 25 at index 3.
Answer:
(a) List[1] = 17
(b) List.extends([8,9,10])
(c) List.pop(0)
(d) List.sort()
(e) List = List * 2
(f) List.insert(3, 25)
Question – 6:
What’s a[1:1] if a is a string of at least two characters? And what if string is shorter?
Answer: If string a is of at least two characters,
>>> a[1:1] => returns empty string ”
if string a is shorter, then also it returns an empty string. Python will not raise any error in this case.
>>> a[1:1] => returns empty string ”
For example:
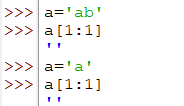
Question – 7:
What are the two ways to add something to a list? How are they different?
Answer: Two ways to add something to list are – (a) append( ) and (b) extend()
append() – append() add an element at the end of the list.
extend() – extend() add list of elements at the end of the list.
Example:
>>>List = [8, 9, 10]
>>> List.append(11)
>>> List
[8, 9, 10, 11]
>>> List.extend([4, 3 , 2])
>>> List
[8, 9, 10, 11, 4, 3, 2]
Question-8:
What are the two ways to remove something from a list? How are they different?
Answer: Two ways to remove something from list are – (a) pop( ) and (b) remove()
pop() = pop() removes an element from a list and return it. By default, it removes the last element from a list.
Syntax:
List.pop() will remove the last element from the list.
List.pop(index_postion) will remove the element of the given index position.
remove() = remove(value) method remove the first occurrence of given item from the list. Takes one argument and does not return anything.
Difference between pop() and remove()
- pop() can work without any argument, while remove() can not.
- pop() removes the element based on index position, while remove() removes the element based on the item value.
- pop() returns the deleted value, while remove() does not return any value.
For example:
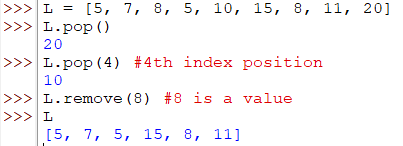
del : del statement can also be used to delete an item or set of items from a list. del statement does not return any value.
- Syntax:
- (i) del List[index] delete one item.
- (ii) del List[start:stop] deletes a set of items comes under the given start to stop-1.
For Example:
Question 9:
What is the difference between a list and a tuple.
Answer: List vs Tuple
List | Tuple |
Lists are mutable. We can change elements of a list in place. | Tuples are not mutable. We can not change elements of a tuple in place. |
The syntax to create list is <list-name> = [value,.....] | The syntax to create tuple is <tuple-name> = (value, ....) |
Lists cannot be used as keys in dictionary. | Tuples can be used as keys in dictionary. |
Lists are slower compared to tuples, dute to mutability. | Tuples are faster compared to lists, due to immutability. |
Question 10:
In the Python shell, do the following:
(i) Define a variable named states that is an empty list.
(ii) Add ‘Delhi’ to the list.
(iii) Now add ‘Punjab’ at the end of the list.
(iv) Define a varible states2 with values ‘Rajasthan’, ‘Gujrat’, and ‘Kerala’.
(v) Add ‘Odisha’ to the beginning of the list states2.
(vi) Add ‘Tripura’ so that it is the third state in the list of states2.
(vii) Add ‘Haryana’ to the list states2 so that it appears before ‘Gujrat’.
(viii) Remove the 5th state from the list states2 and print the state’s name.
Answer:
- >>> states = [] or states = list()
- >>> states.append(‘Delhi’)
- >>> states.append(‘Punjab’)
- >>>states2 =[‘Rajasthan’, ‘Gujrat’, ‘Kerala’]
- >>> states2.insert(0, ‘Odisha’)
- >>> states2.insert(2, ‘Tripura’)
- >>> states2.insert( states2.index(‘Gujrat’), ‘Haryana’)
- >>> states2.pop(4)
>>> print(states2)
Question 11:
Discuss the utility and significance of Tuples, briefly.
Answer: Tuples are immutable data type, which does not allow to make changes in place. It is mainly used with a constant list. Constant list means which values can not be changed by anyone either intentionally or accidentally.
Question 12:
If a is (1, 2, 3)
- (a) What is the difference between a * 3 and (a, a, a) ?
- (b) is a * 3 equivalent to a+a+a ?
- (c) what is the meaning of a[1:1] ?
- (d) What is the difference between a[1:2] and a[1:1] ?
Answer:
(a) a * 3 will create a tuple having values (1, 2, 3, 1, 2, 3, 1, 2, 3). * is the replication operator.
(a, a, a) will creates nested tuples having values ((1, 2, 3), (1, 2, 3), (1, 2, 3))
(b) Yes, both a * 3 and a + a + a will result in (1, 2, 3, 1, 2, 3, 1, 2, 3).
(c) It returns an empty tuple () . It means slice the tuple from index 1 to 0. This is an invalid range.
(d) Both are tuple slicing statement, which returns tuple slice from start to stop-1 index position.
a[1:2] returns (2,). It will returns from index 1 to 1,
a[1:1] returns (). It try to return from index 1 to 0, that is an invalid slice.
Question 13:
What is the difference between (30) and (30,) ?
Answer: (30) is an integer data type while (30,) is a tuple data type.
Question 14:
Write a Python statement to declare a Dictionary named ClassRoll with Keys as 1, 2, 3 and corresponding values as ‘Reena’, ‘Rakesh’, ‘Zareen’ respectively. [CBSE 2020C]
Answer: ClassRoll = {1 : ‘Reena’, 2 : ‘Rakesh’, 3 : ‘Zareen’}
Question 15:
Why is a dictionary termed as an unordered collection of objects ?
Answer: Dictionary stores the value as {Key:Value} pair. It does not have any index position like string, list, or tuples. Because all values of the dictionary are linked (accessed) through the keys of the dictionary. So that the {key: value} pair of dictionaries can be stored in any order inside the computer memory. That’s why it is called an unordered collection of objects.
Question 16:
What type of objects can be used as keys in dictionaries ?
Answer: Keys of a dictionary must be of immutable data types such as –
- Number (Integers, Float, Complex),
- String, and
- Tuple (containing only immutable entries.
Question 17:
Though tuples are immutable type, yet they cannot always be used as keys in a dictionary. What is the condition to use tuples as a key in a dictionary?
Answer: For a tuple to be used as a key in a dictionary, all its elements must be immutable as well. If a tuple contains mutable elements, such as lists, sets, or other dictionaries, it cannot be used as a key in a dictionary.
Question – 18:
Dictionary is a mutable type, which means you can modify its contents? What all is modifiable in a dictionary? Can you modify the keys of a dictionary?
Answer: The dictionary is a mutable data type in Python. It means you can modify only values (content) of dictionary.
Values of key-value pairs are modifiable in dictionary. New key-value pairs can also be added to an existing dictionary and existing key-value pairs can be removed.
However, The keys of the dictionary can not be modified, because only value of dictionary is mutable is keys is not.
Instead we can add new key:value pair and delete the existing key:value pair.
Question – 19:
How is del D and del D[<key>] different from one another if D is a dictionary ?
Answer: del D
deletes the entire dictionary D. After executing del D
, the variable D is no longer defined, and any attempt to access D
will result in a NameError
.del D[<key>]
deletes the key-value pair associated with the specified key from the dictionary D
. After executing del D[<key>]
, the dictionary D
still exists, but the specified key and its corresponding value are removed from the dictionary.
For example:
D = {1 : 10, 2 : 25, 3 : 30, 4 : 40}
print(D)
del D[3]
print(D)
del D
print(D)
Output:
![Python Revision Tour II Difference between del D and del D[key].](https://anjeevsinghacademy.com/wp-content/uploads/2024/06/image.png)
Question – 20:
Create a dictionary named D with three entries, for keys ‘a’, ‘b’ and ‘c’. What happens if you try to index a nonexistent key (D[‘d’]) ? What does python do if you try to assign to a nonexistent key d.
(e.g., D[‘d’] = ‘spam’) ?
Answer: The dictionary D does not contain the key ‘d’, so when you try to access D[‘d’], it results in a KeyError, because key does not exists.
D[‘d’] = ‘spam’
If we try to assign a value to a nonexistent key ‘d’ in a dictionary, Python will create that key-value pair {‘d’:’spam’} in the dictionary.
For example:
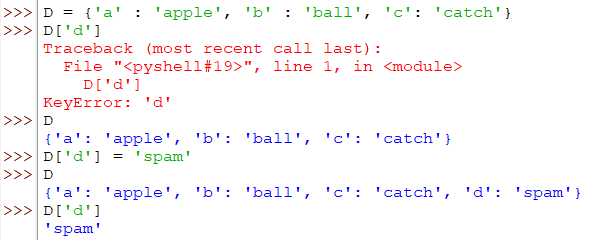
Question 21:
What is sorting ? Name some popular sorting techniques.
Answer: Sorting refers to arranging elements of a sequence in a specific order — ascending or descending.
Sorting Techniques are as follows :
- Bubble Sort
- Insertion Sort
- Selection Sort
- Quick Sort
Question 22:
Discuss Bubble sort and Insertion sort techniques.
Answer:
Bubble Sort :
In Bubble sort, the adjoining values are compared and exchanged if they are not in proper order. This process is repeated until the entire array is sorted.
Insertion Sort :
Insertion sort is a sorting algorithm that builds a sorted list one element at a time from the unsorted list by inserting the element at its correct position in the sorted list.
Type B: Application-Based Questions – Python Revision Tour II
Question 1:
What will be the output produced by following code fragments?
Question 1 (a): Find the output.
y = str(123)
x = "hello" \* 3
print(x, y)
x = "hello" + "world"
y = len(x)
print(y, x)
Answer:
hellohellohello 123
10 helloworld
Question 1 (b): Find the output.
x = "hello" + \
"to Python" + \
"world"
for char in x :
y = char
print(y, ':', end=" ")
Answer:
h : e : l : l : o : t : o : : P : y : t : h : o : n : w : o : r : l : d :
Question 1 (c): Find the output.
x = "hello world"
print(x[:2], x[:-2], x[-2:])
print(x[6], x[2:4])
print(x[2:-3], x[-4:-2])
Answer:
he hello wor ld
w ll
llo wo or
Question 2:
Write a short Python code segment that adds up the lengths of all the words in a list and then prints the average (mean) length.
word_list = eval(input("Enter a list of words: "))
total_length = 0
for word in word_list:
total_length += len(word)
average_length = total_length / len(word_list)
print("Average length of words:", average_length)
Answer:
Enter a list of words: ["new delhi", "patna", "hisar", "lucknow"]
Average lengh of words: 6.5
Question 3:
Predict the output of the following code snippet.
a = [1, 2, 3, 4, 5]
print(a[3:0:-1])
Answer:
[4, 3, 2]
Question 4 (a):
Predict the output of the following code snippet?
arr = [1, 2, 3, 4, 5, 6]
for i in range(1, 6):
arr[i - 1] = arr[i]
for i in range(0, 6):
print(arr[i], end = "")
Answer:
Output :
234566
Question 4 (b):
Predict the output of the following code snippet.
Numbers = [9, 18, 27, 36]
for Num in Numbers :
for N in range(1, Num % 8) :
print(N, "#", end=" ")
print( )
Answer:
Output
1 #
1 # 2 #
1 # 2 # 3 #
Question 5(a):
Find the errors. State reasons.
t = (1, "a", 9.2)
t[0] = 6
Answer: t[0] = 6 will raise a TypeError as tuples are immutable. t is a tuple, so that its element cannot be changed after creation.
Question 5(b):
Find the errors. State reasons.
t = [1, "a", 9.2]
t[0] = 6
Answer: No Error.
List is a mutable type, so t[0] = 6 is a valid statement. We can change the value of list after creation.
Question 5(c):
Find the errors. State reasons.
t = [1, "a", 9.2]
t[4] = 6
Answer: t[4] = 6, will raise an error IndexError – list index out of range. It means t[4]= 6 is trying to change the value at index 4, but it is outside the current range of list t. As t has only three elements so valid indexes are 0, 1, 2 only.
Question 5(d):
Find the errors. State reasons.
t = 'hello'
t[0] = "H"
Answer: t[0] = “H” raise a TypeError: ‘str’ object does not support item assignment. t is a string, in Python string is immutable. Due to immutability, we cannot change individual elements (characters) in a string after creation.
Question 5(e):
Find the errors. State reasons.
for Name in [Amar, Shveta, Parag]
IF Name[0] = 'S':
print(Name)
Answer: This code has the following errors –
Line 1 – Undefined Symbol – Amar, Shveta, and Parag. All elements must be enclosed in quotes, because they are strings.
Line 2 – Undefined Symbol IF, IF statement must be in lowercase if.
Line 2 – Invalid operator =, it should be ==.
= is used for assignment while == is used for comparison.
Question 6:
Assuming words is a valid list of words, the program below tries to print the list in reverse. Does it have an error? If so, why? (Hint. There are two problems with the code.)
for i in range(len(words), 0, -1):
print(words[i], end=' ')
Answer:
There are two issue in range(len(words), 0, -1)
:
- The start index
len(words)
is invalid for the listwords
as it will have indexes from 0 tolen(words) - 1
. - The end index being 0 means that the last element of the list is missed as the list will be iterated till index 1 only.
Corrected Code :
for i in range(len(words)-1, -1, -1):
print(words[i], end=' ')
Question 7:
What would be the output of following code if ntpl = (“Hello”, “Nita”, “How’s”, “life ?”) ?
(a, b, c, d) = ntpl
print("a is:", a)
print("b is:", b)
print("c is:", c)
print("d is:", d)
ntpl = (a, b, c, d)
print(ntpl[0][0] + ntpl[1][1], ntpl[1])
Answer: Output-
a is: Hello
b is: Nita
c is: How's
d is: life ?
Hi Nita
Question 8:
What will be the output of the following code ?
tuple_a = 'a', 'b'
tuple_b = ('a', 'b')
print (tuple_a == tuple_b)
(a) 0 (b) 1 (c) False (d) True
Answer: Output-
True
Reason:
In Python, tuples can be created with or without parentheses. In Python, comma-separated values are also known as tuples. That’s why, print(tuple_a == tuple_b), prints True
Question 9:
What will be the output of the following code snippet ?
rec = {"Name" : "Python", "Age" : "20", "Addr" : "NJ", "Country" : "USA"}
id1 = id(rec)
del rec
rec = {"Name" : "Python", "Age" : "20", "Addr" : "NJ", "Country" : "USA"}
id2 = id(rec)
print(id1 == id2)
(a) True (b) False (c) 1 (d) Exception
Answer: (a) True
Reason:
In the given python code snippet, id1
and id2
will point to two different objects in memory as del rec
deleted the original dictionary whose id is stored in id1
and created a new dictionary with the same contents storing its id in id2
. However, id1 == id2
will compare the contents of the two dictionaries pointed to by id1
and id2
. As contents of both the dictionaries are same hence it returns True
.
If in this code we add another line print(id1 is id2)
then this line will print False
as id1
and id2
point to two different dictionary objects in memory.
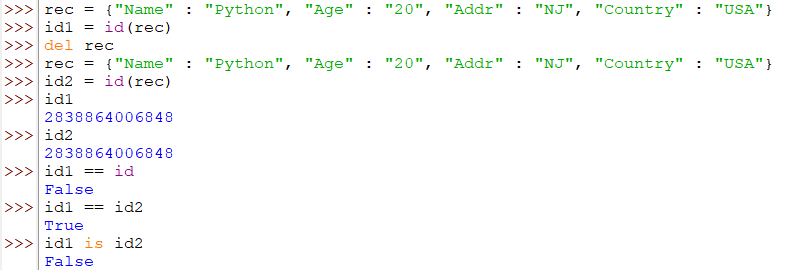
Question 10:
Write the output of the code given below :
my_dict = {"name" : "Aman", "age" : 26}
my_dict['age'] = 27
my_dict['address'] = "Delhi"
print(my_dict.items())
Answer: Output
dict_items([('name', 'Aman'), ('age', 27), ('address', 'Delhi')])
Question – 11:
Write a method to display the elements of the list thrice if it is a number and display the element terminated with ‘#’ if it is not a number.
For example, if the content of list is as follows :
List = ['41', 'DROND', 'GIRIRAJ', '13', 'ZARA']
The output should be
414141
DROND#
GIRIRAJ#
131313
ZAR#
Answer: Python Method
def printList(List):
for val in List:
if val.isdigit():
print(val*3)
else:
print(val,'#', sep='')
List = ['41', 'DROND', 'GIRIRAJ', '13', 'ZARA']
printList(List)
Output:
414141
DROND#
GIRIRAJ#
131313
ZARA#
Question 12: Name the function/method required to
(i) check if a string contains only uppercase letters.
(ii) gives the total length of the list.
Answer: (i) Check if a string contains only uppercase letters – isupper()
(ii) gives the total length of the list – len()
Question 13:
What will be the output of the following code snippet ?
my_dict = {}
my_dict[(1,2,4)] = 8
my_dict[(4,2,1)] = 10
my_dict[(1,2)] = 12
sum = 0
for k in my_dict:
sum += my_dict[k]
print(sum)
print(my_dict)
Answer: Output
30
{(1, 2, 4): 8, (4, 2, 1): 10, (1, 2): 12}
Type C: Programming Practice / Knowledge-Based Questions – Python Revision Tour II
Question – 1: Phone Number Format Validation
Write a program that prompts for a phone number of 10 digits and two dashes, with dashes after the area code and the next three numbers. For example, 017-555-1212 is a legal input.
Display if the phone number entered is valid format or not and display if the phone number is valid or not (i.e., contains just the digits and dash at specific places).
Answer:
#Que - 1 : Phone Number Format Validaion
phoneNo = input("Enter Phone Number xxx-xxx-xxxx format : ")
if phoneNo.count('-') == 2 and len(phoneNo) == 12 \
and phoneNo[3] == '-' and phoneNo[7] == '-' and \
phoneNo[0:3].isdigit() and phoneNo[4:7].isdigit()\
and phoneNo[8:].isdigit():
print("You entered a VALID phone number", phoneNo)
else:
print("Phone number is INVALID", phoneNo)
Output:
Sample Run-1 :
Enter Phone Number xxx-xxx-xxxx format : 123-456-7890
You entered a VALID phone number 123-456-7890
Sample Run-2 :
Enter Phone Number xxx-xxx-xxxx format : 12-3655-4598
Phone number is INVALID 12-3655-4598
Question – 2:
Write a program that should prompt the user to type some sentence(s) followed by “enter”. It should then print the original sentence(s) and the following statistics relating to the sentence(s):
- Number of words.
- Number of characters (including white-space and punctuation).
- Percentage of characters that are alpha-numeric.
Hints:
- Assume any consecutive sequence of non-blank characters in a word.
Answer:
#Que - 2 : Enter Sentence and Print statistics relating to sentence.
sentence = input("Enter a sentence :")
words = len(sentence.split())
nochars = len(sentence)
count = 0
for char in sentence:
if char.isalnum():
count +=1
alnumper = (count / nochars) * 100
print("Number of Words : ", words)
print("Number of Characters : ", nochars)
print("Percentage of characters that are alpha numeric :", alnumper)
Output:
Enter a sentence: Your roll number is 10. You scored 25 out of 30.
Number of Words: 11
Number of Characters: 48
Percentage of characters that are alpha numeric: 75.0
Question – 3:
Write a program that takes any two lists L and M of the same size and adds their elements together to form a new list N whose elements are sums of the corresponding elements in L and M. For instance, if L = [3, 1, 4] and M = [1, 5, 9], then N should equal [4, 6, 13].
Answer:
#Que 3 - Sum of two lists element wise
L = [3, 1, 4] #List 1
M = [1, 5, 9] #List 2
N = [] #Empty List
for i in range(3):
N.append(L[i] + M[i]) #Adding element and appending
print("Sum of two Lists :", N)
Output:
Sum of two Lists : [4, 6, 13]
Question – 4: Rotate the element of the list i.e. one position right shift
Write a program that rotates the elements of a list so that the element at the first index moves to the second index, the element in the second index moves to the third index, etc., and the element in the last index moves to the first index.
Answer:
#Que 4 - Rotate the element of List i.e. Right Shift
L = [5, 3, 2, 1, 9]
print("List Before Shifting")
print(L)
temp = L[len(L)-1] #last element
for i in range(len(L)-2,-1,-1):
L[i+1] = L[i] #Copy current element to next position
L[0] = temp #Copy the last element at 0 position
print("List After Shifting")
print(L)
Output:
List Before Shifting
[5, 3, 2, 1, 9]
List After Shifting
[9, 5, 3, 2, 1]
Question – 5:
Write a short Python code segment that prints the longest word in a list of words.
Answer:
#Que 5 - Print longest words in a list of words.
string = input("Enter a sentence : ")
wordList = string.split()
print("List of words are =>", wordList, sep='\n')
long_word = ''
for word in wordList:
if len(word) > len(long_word):
long_word = word
print("The longest word in the List is => ", long_word)
Output:
Enter a sentence: Hello my dear students, you are doing excellent in your study.
Original List :
['Hello', 'my', 'dear', 'students,', 'you', 'are', 'doing', 'execellent', 'in', 'your', 'study.']
The longest word in the List is => excellent
script async=”” src=”https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js?client=ca-pub-7862195577776589″ crossorigin=”anonymous”>
Question – 6:
Write a program that creates a list of all the integers less than 100 that are multiples of 3 or 5.
Answer:
#Que 6 - Lists of all the integers less than 100 that are multiples of 3 or 5.
L = []
for i in range(1, 100):
if i % 3 == 0 or i % 5 == 0:
L.append(i)
print("List are => ", L, sep = '\n')
Output:
List are =>
[3, 5, 6, 9, 10, 12, 15, 18, 20, 21, 24, 25, 27, 30, 33, 35, 36, 39, 40, 42, 45, 48, 50, 51, 54, 55, 57, 60, 63, 65, 66, 69, 70, 72, 75, 78, 80, 81, 84, 85, 87, 90, 93, 95, 96, 99]
Question 7:
Define two variables first and second so that first = “Jimmy” and second = “Johny”. Write a short python code segment that swaps the values assigned to these two variables and prints the results.
first = "Jimmy"
second = "Johny"
temp = first
first = second
second = temp
print("first =", first)
print("second =", second)
first = Johny
second = Jimmy
Write a python program that creates a tuple storing first 9 terms of Fibonacci series.
lst = [0,1]
a = 0
b = 1
c = 0
for i in range(7):
c = a + b
a = b
b = c
lst.append(c)
tup = tuple(lst)
print("9 terms of Fibonacci series are:", tup)
9 terms of Fibonacci series are: (0, 1, 1, 2, 3, 5, 8, 13, 21)
Create a dictionary whose keys are month names and whose values are the number of days in the corresponding months.
(a) Ask the user to enter a month name and use the dictionary to tell them how many days are in the month.
(b) Print out all of the keys in alphabetical order.
(c) Print out all of the months with 31 days.
(d) Print out the (key-value) pairs sorted by the number of days in each month.
days_in_months = {
"january":31,
"february":28,
"march":31,
"april":30,
"may":31,
"june":30,
"july":31,
"august":31,
"september":30,
"october":31,
"november":30,
"december":31
}
m = input("Enter name of month: ")
if m not in days_in_months:
print("Please enter the correct month")
else:
print("There are", days_in_months[m], "days in", m)
print("Months in alphabetical order are:", sorted(days_in_months))
print("Months with 31 days:", end=" ")
for i in days_in_months:
if days_in_months[i] == 31:
print(i, end=" ")
day_month_lst = []
for i in days_in_months:
day_month_lst.append([days_in_months[i], i])
day_month_lst.sort()
month_day_lst =[]
for i in day_month_lst:
month_day_lst.append([i[1], i[0]])
sorted_days_in_months = dict(month_day_lst)
print()
print("Months sorted by days:", sorted_days_in_months)
Enter name of month: may
There are 31 days in may
Months in alphabetical order are: ['april', 'august', 'december', 'february', 'january', 'july', 'june', 'march', 'may', 'november', 'october', 'september']
Months with 31 days: january march may july august october december
Months sorted by days: {'february': 28, 'april': 30, 'june': 30, 'november': 30, 'september': 30, 'august': 31, 'december': 31, 'january': 31, 'july': 31, 'march': 31, 'may': 31, 'october': 31}
Write a function called addDict(dict1, dict2) which computes the union of two dictionaries. It should return a new dictionary, with all the items in both its arguments (assumed to be dictionaries). If the same key appears in both arguments, feel free to pick a value from either.
def addDict(dict1, dict2):
union_dict = {}
for key, value in dict1.items():
union_dict[key] = value
for key, value in dict2.items():
union_dict[key] = value
return union_dict
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
result = addDict(dict1, dict2)
print("Union of dict1 and dict2:", result)
Output:
Union of dict1 and dict2: {'a': 1, 'b': 3, 'c': 4}
Write a program to sort a dictionary’s keys using Bubble sort and produce the sorted keys as a list.
my_dict = eval(input("Enter the dictionary: "))
keys = list(my_dict.keys())
l = len(keys)
for i in range(l):
for j in range(0, l - i - 1):
if keys[j] > keys[j + 1]:
keys[j], keys[j + 1] = keys[j + 1], keys[j]
print("Sorted keys:",keys)
Enter the dictionary: {'c':10, 'f':87, 'r':23, 'a':5}
Sorted keys: ['a', 'c', 'f', 'r']
Question 12:
Write a program to sort a dictionary’s values using Bubble sort and produce the sorted values as a list.
Answer:
my_dict = eval(input("Enter the dictionary: "))
values = list(my_dict.values())
l = len(values)
for i in range(l):
for j in range(0, l - i - 1):
if values[j] > values[j + 1]:
# Swap values
values[j], values[j + 1] = values[j + 1], values[j]
print("Sorted values:", values)
Enter the dictionary: {'w':34, 'a':5, 'g':45, 't':21}
Sorted values: [5, 21, 34, 45]