Type C: Practical/Knowledge-Based Questions
7. The score of a team in 5 IPL matches is available to you. Write a program to create a pie chart from this data, showing the last match’s performance as a wedge.
Ans:
import pandas as pd
import matplotlib.pyplot as plt
IPL = {‘CSK’:[250,350,216,315,278],
‘RR’:[298,247,231,264,198],
‘DDW’:[310,254,279,249,233],
‘RCB’:[216,239,258,365,347]}
dfIPL = pd.DataFrame(IPL, index=range(1,6))
print(dfIPL)
plt.pie(dfIPL.loc[5].values, labels=dfIPL.columns, autopct=”%2d%%”)
plt.title(“Last IPL Match – Wedge”)
plt.show()
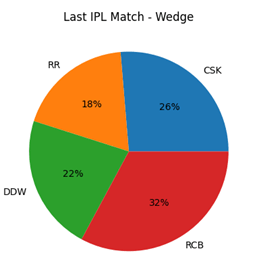
8. The prices of a stock for 3 months are given. Write a program to show the variations in prices for each month by 3 lines on same line chart. Make sure to add legends and labels. Show grid also.
Ans:
import pandas as pd
import matplotlib.pyplot as plt
month = [‘Jan’, ‘Feb’, ‘Mar’]
janPrice = [25,36,21,26,39]
febPrice = [28,40,38,42,40]
marPrice = [42,46,40,39,48]
x = [1,2,3,4,5]
plt.plot(x, janPrice, label=’Jan’)
plt.plot(x, febPrice, label=’Feb’)
plt.plot(x, marPrice, label=’Mar’)
plt.title(“Price Variations”)
plt.xlabel(“Number”)
plt.ylabel(“Price”)
plt.grid(True)
plt.legend()
plt.show()

9. A distribution data stores about 1000 random numbers. Write a program to create a scatter chart from this data with varying point sizes.
Ans:
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
x = range(1,1001)
rndnumber = np.random.randint(10,500,1000)
size = np.random.randint(50,100,1000)
plt.scatter(x, rndnumber, s=size)
plt.show()
