Type C: Practical/Knowledge-Based Questions
12. Create an ndarray containing 16 values and then plot this array along with dataset of previous question in same histogram
(a) normal histograms
(b) cumulative histograms
(c) horizontal histograms
Ans:
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
measurement = [78,72,69,81,63,67,65,75,
79,74,71,83,71,79,80,69]
arr = np.random.randint(60,90, size=(16,))
#(a) normal histograms
plt.hist([measurement, arr], label=[‘measurement’, ‘array’])
plt.legend()
plt.show()

#(b) cumulative histograms
plt.hist([measurement,arr], cumulative=True, label=[‘measurement’, ‘array’])
plt.legend()
plt.show()

#(c) horizontal histograms
plt.hist([measurement, arr], orientation=’horizontal’, label=[‘measurement’, ‘array’])
plt.legend()
plt.show()

13. Out of above-plotted histograms, which ones can be used for creating frequency polygons? Can you draw frequency polygons from all the above histograms?
Ans: The step type normal histogram can be used for creating frequency polygon.
There is no direct method in PyPlot, to make the frequency polygon. But with the help of hist( ) and plot( ) you can try to make the frequency polygon.
14. Create/draw a frequency polygon from the data used in the above questions.
Ans:
Step – 1: Creating Histogram chart – step typed
import pandas as pd
import matplotlib.pyplot as plt
measurement = [78,72,69,81,63,67, 65,75,79,74,71,83,71,79,80,69]
plt.hist(measurement, histtype=’step’)
plt.show()
Step – 2: Plot a best fit line manually.
2.1 Mark midpoints of every bin.
2.2 Join midpoints of every bin with a line
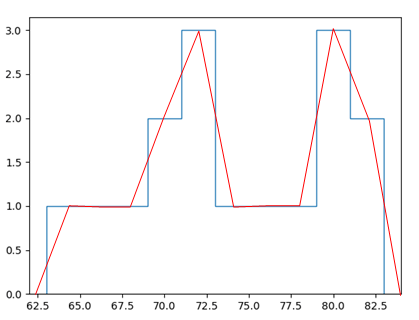